Radio
Radio settings provide a set of mutually exclusive options for users to choose from. This is ideal for settings where users need to select one option from a small set of choices.
Basic Usage
import { useMMAppSetting } from '@machinemetrics/mm-react-tools';
function MyWidget() {
useMMAppSetting('thresholdEnabled', {
label: 'Utilization Goal',
type: 'radio',
options: [
{ value: 'default', label: 'Default' },
{ value: 'custom', label: 'Custom' },
],
default: 'default',
});
}
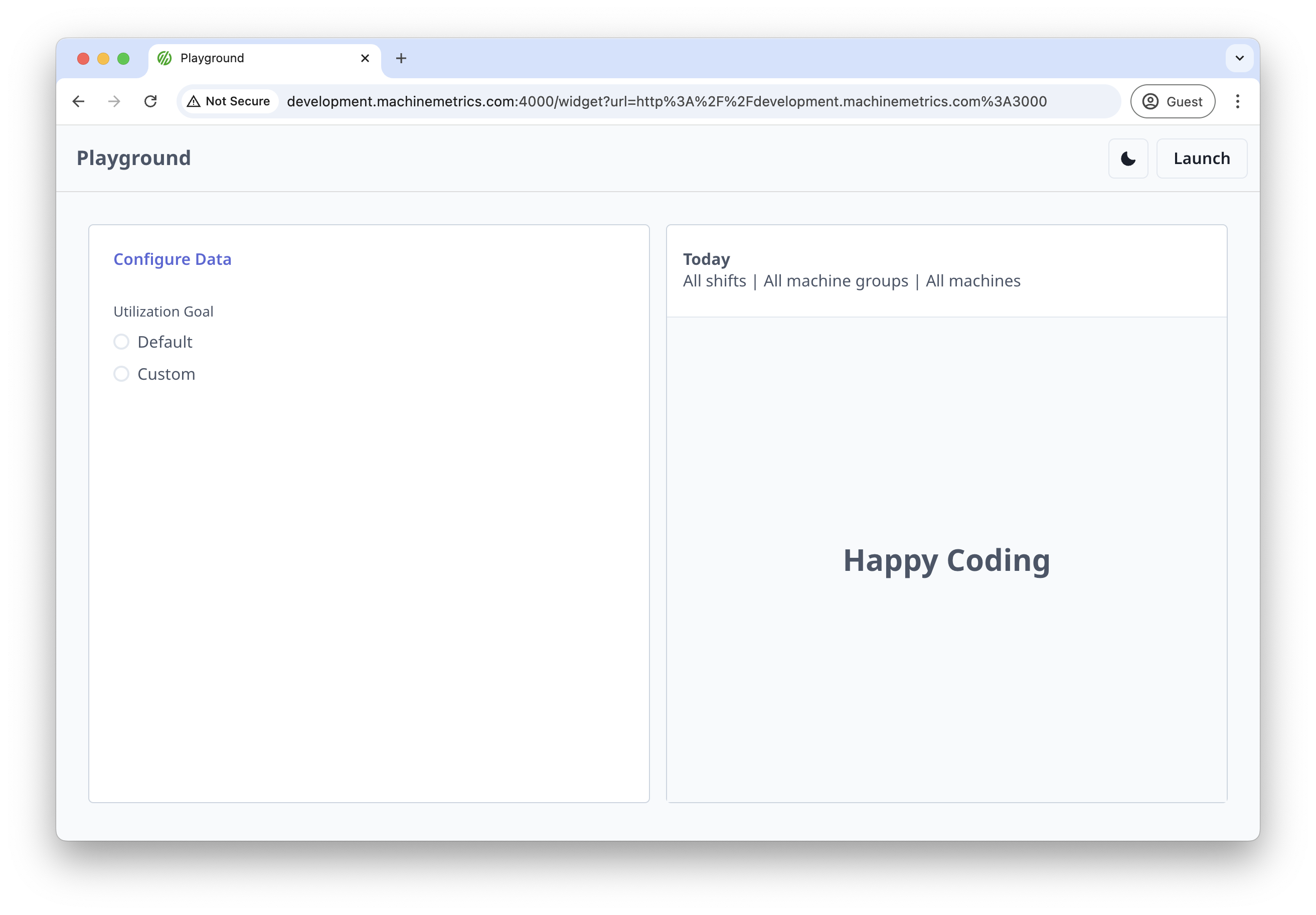
Properties
Property | Type | Description |
---|---|---|
label | string | Display label for the radio group |
type | "radio" | Must be set to "radio" |
options | array/function | Array of option objects or function returning options |
default | string | Default selected value |
isRequired | boolean | Whether a selection is required |
helperText | string | Help text displayed below the radio group |
validate | function | Validation function for the selected value |
Dynamic Options
You can provide options dynamically using a function:
useMMAppSetting('aggregationType', {
label: 'Aggregation Type',
type: 'radio',
options: () => {
// Example of dynamic options based on available metrics
return availableMetrics.map((metric) => ({
label: metric.name,
value: metric.id,
}));
},
});
Examples
Basic Radio Selection
useMMAppSetting('displayMode', {
label: 'Display Mode',
type: 'radio',
default: 'chart',
options: [
{ value: 'chart', label: 'Chart View' },
{ value: 'table', label: 'Table View' },
{ value: 'list', label: 'List View' },
],
});
Radio Selection with Dependencies
useMMAppSetting(
'thresholdEnabled',
{
label: 'Utilization Goal',
type: 'radio',
options: () => {
return [
{ value: 'default', label: 'Default' },
{ value: 'custom', label: 'Custom' },
];
},
default: 'default',
},
[],
);
Radio Selection with Validation
useMMAppSetting('dataSource', {
label: 'Data Source',
type: 'radio',
isRequired: true,
helperText: 'Select the source of data for this widget',
validate: ({ value }) => {
if (!['live', 'historical', 'hybrid'].includes(value)) {
return { message: 'Invalid data source selected' };
}
return null;
},
options: [
{ value: 'live', label: 'Live Data' },
{ value: 'historical', label: 'Historical Data' },
{ value: 'hybrid', label: 'Hybrid (Live + Historical)' },
],
});
Radio Selection with Dynamic Options
useMMAppSetting('metricType', {
label: 'Metric Type',
type: 'radio',
options: () => {
// Example of dynamic options based on available metric types
return [
{ value: 'oee', label: 'OEE' },
{ value: 'utilization', label: 'Utilization' },
{ value: 'availability', label: 'Availability' },
{ value: 'quality', label: 'Quality' },
].filter((option) => availableMetricTypes.includes(option.value));
},
default: 'oee',
});